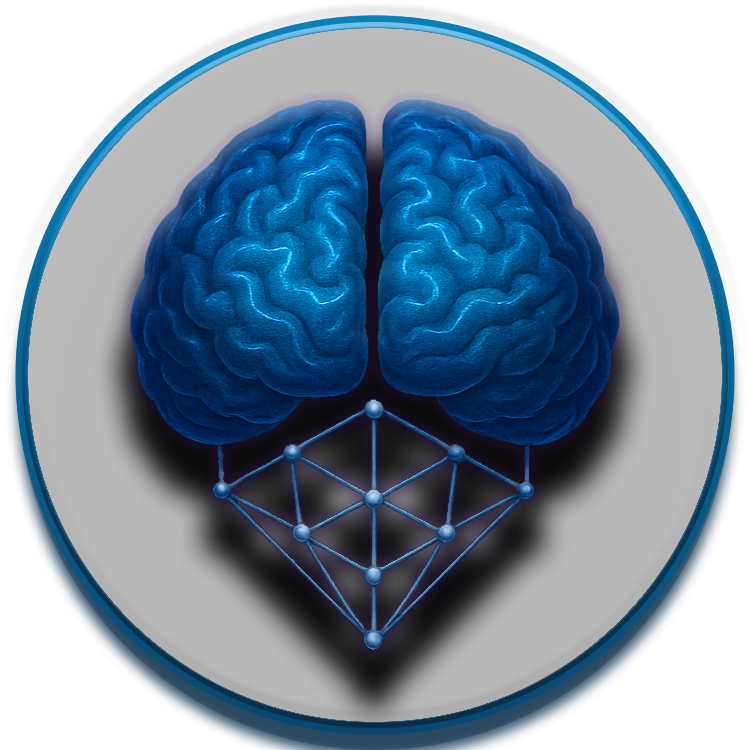
Development Workflow¶
This document outlines the development workflow for the NeuroCognitive Architecture (NCA) project. It provides guidelines for code development, testing, review, and deployment processes to ensure consistent, high-quality contributions across the team.
Table of Contents¶
- Development Environment Setup
- Branching Strategy
- Development Lifecycle
- Code Quality Standards
- Testing Guidelines
- Code Review Process
- Continuous Integration
- Deployment Process
- Documentation Requirements
- Issue and Bug Tracking
- Release Management
Development Environment Setup¶
Prerequisites¶
- Python 3.12+
- Docker and Docker Compose
- Git
- Poetry (for dependency management)
- Make (optional, for running Makefile commands)
Initial Setup¶
-
Clone the repository:
-
Set up the environment:
-
Install dependencies:
-
Set up pre-commit hooks:
-
Start the development environment:
Branching Strategy¶
We follow a Git Flow-inspired branching strategy:
main
: Production-ready codedevelop
: Integration branch for featuresfeature/*
: New features or enhancementsbugfix/*
: Bug fixeshotfix/*
: Urgent fixes for productionrelease/*
: Release preparation
Branch Naming Conventions¶
- Feature branches:
feature/issue-number-short-description
- Bug fix branches:
bugfix/issue-number-short-description
- Hotfix branches:
hotfix/issue-number-short-description
- Release branches:
release/vX.Y.Z
Development Lifecycle¶
- Issue Creation: All work begins with an issue in the issue tracker
- Branch Creation: Create a branch from
develop
using the appropriate naming convention - Development: Implement the feature or fix
- Testing: Write and run tests locally
- Code Review: Submit a pull request and address feedback
- Integration: Merge into
develop
after approval - Release: Merge into
main
as part of a release - Deployment: Deploy to production
Commit Guidelines¶
- Use conventional commit messages:
feat:
for new featuresfix:
for bug fixesdocs:
for documentation changesstyle:
for formatting changesrefactor:
for code refactoringtest:
for adding or modifying tests-
chore:
for maintenance tasks -
Include the issue number in the commit message:
feat(memory): Implement working memory system (#123)
Code Quality Standards¶
Code Style¶
- Follow PEP 8 for Python code
- Use type hints for all function parameters and return values
- Maximum line length: 100 characters
- Use descriptive variable and function names
Linting and Formatting¶
The following tools are configured in the pre-commit hooks:
- Black for code formatting
- isort for import sorting
- Flake8 for linting
- mypy for type checking
Run checks manually:
Testing Guidelines¶
Test Coverage Requirements¶
- Minimum test coverage: 80%
- All core functionality must have unit tests
- Integration tests for component interactions
- End-to-end tests for critical user flows
Running Tests¶
# Run all tests
poetry run pytest
# Run with coverage report
poetry run pytest --cov=neuroca
# Run specific test file
poetry run pytest tests/path/to/test_file.py
Code Review Process¶
- Pull Request Creation:
- Create a pull request from your feature branch to
develop
- Fill out the PR template with details about the changes
-
Link the relevant issue(s)
-
Review Requirements:
- At least one approval from a core team member
- All CI checks must pass
-
No unresolved comments
-
Merge Process:
- Squash and merge for feature branches
- Rebase and merge for hotfixes
Continuous Integration¶
Our CI pipeline includes:
- Linting and code style checks
- Unit and integration tests
- Test coverage reporting
- Security scanning
- Build validation
Deployment Process¶
Environments¶
- Development: Automatic deployment from the
develop
branch - Staging: Manual deployment from release branches
- Production: Manual deployment from the
main
branch
Deployment Steps¶
- Create a release branch from
develop
:release/vX.Y.Z
- Perform final testing and fixes on the release branch
- Create a pull request to merge into
main
- After approval, merge to
main
and tag the release - Deploy to production using the CI/CD pipeline
Documentation Requirements¶
Code Documentation¶
- All modules, classes, and functions must have docstrings
- Use Google-style docstrings format
- Document parameters, return values, and exceptions
Project Documentation¶
- Architecture documentation in
/docs/architecture/
- API documentation in
/docs/api/
- User guides in
/docs/guides/
- Development documentation in
/docs/development/
Issue and Bug Tracking¶
- Use GitHub Issues for tracking bugs and features
- Label issues appropriately (bug, enhancement, documentation, etc.)
- Include steps to reproduce for bug reports
- Link issues to relevant pull requests
Release Management¶
Versioning¶
We follow Semantic Versioning (SemVer): - Major version: Incompatible API changes - Minor version: Backward-compatible new features - Patch version: Backward-compatible bug fixes
Release Notes¶
- Document all significant changes in CHANGELOG.md
- Categorize changes (Added, Changed, Fixed, Removed)
- Include contributor acknowledgments
Release Process¶
- Update version numbers in relevant files
- Update CHANGELOG.md with release notes
- Create a release branch:
release/vX.Y.Z
- Create a pull request to
main
- After merging, tag the release in Git
- Create a GitHub Release with release notes
Troubleshooting¶
Common Issues¶
- Environment setup problems: Check the
.env
file and Docker logs - Dependency conflicts: Update Poetry lock file with
poetry update
- Pre-commit hook failures: Run hooks manually to see detailed errors
Getting Help¶
- Check the project wiki for common solutions
- Ask in the development Slack channel
- Create an issue with the "question" label
This workflow document is a living guide and will be updated as our processes evolve. All team members are encouraged to suggest improvements through pull requests.