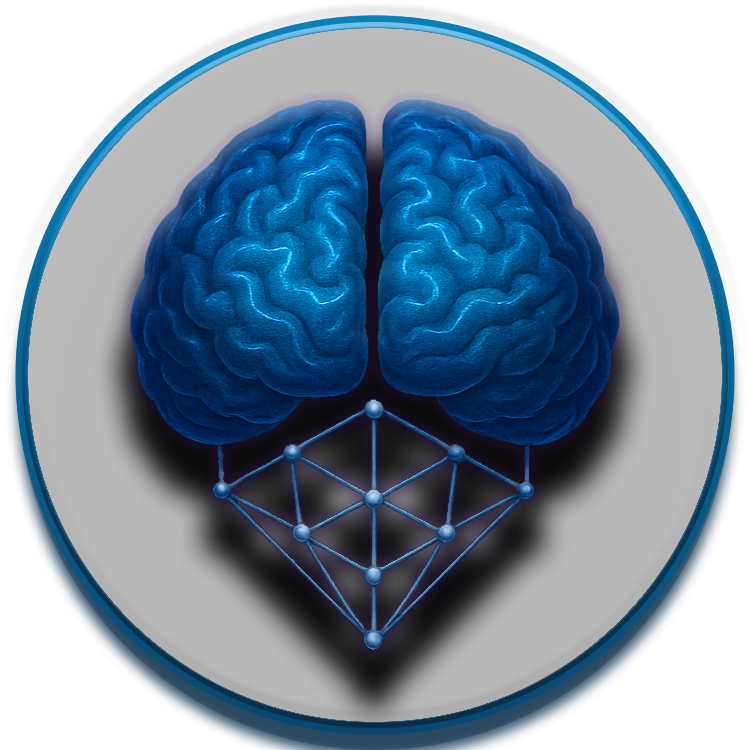
NeuroCognitive Architecture (NCA) Development Standards¶
Table of Contents¶
- Introduction
- Code Style and Formatting
- Documentation Standards
- Testing Requirements
- Security Guidelines
- Performance Considerations
- Version Control Practices
- Code Review Process
- Dependency Management
- Error Handling
- Logging Standards
- Accessibility
- Internationalization and Localization
- Continuous Integration and Deployment
- Monitoring and Observability
Introduction¶
This document outlines the development standards for the NeuroCognitive Architecture (NCA) project. Adhering to these standards ensures code quality, maintainability, and consistency across the codebase. All contributors are expected to follow these guidelines.
Code Style and Formatting¶
Python¶
- Follow PEP 8 style guide
- Use Black for code formatting with a line length of 88 characters
- Use isort for import sorting
- Use flake8 for linting
- Use type hints for all function parameters and return values
# Good example
def process_memory_item(item: MemoryItem, context: Context) -> ProcessingResult:
"""Process a memory item within the given context.
Args:
item: The memory item to process
context: The context in which to process the item
Returns:
The result of processing the memory item
Raises:
InvalidMemoryError: If the memory item is invalid
"""
if not item.is_valid():
raise InvalidMemoryError(f"Invalid memory item: {item.id}")
result = context.process(item)
return result
JavaScript/TypeScript (for web interfaces)¶
- Use ESLint with the Airbnb configuration
- Use Prettier for code formatting
- Use TypeScript for all new code
- Maximum line length of 100 characters
General¶
- Use meaningful variable and function names that describe their purpose
- Keep functions small and focused on a single responsibility
- Avoid deep nesting of control structures
- Use constants for magic numbers and strings
- Follow the DRY (Don't Repeat Yourself) principle
Documentation Standards¶
Code Documentation¶
- All modules, classes, and functions must have docstrings
- Use Google style docstrings for Python
- Document parameters, return values, and exceptions
- Include examples for complex functions
- Document any non-obvious behavior or edge cases
Project Documentation¶
- Architecture decisions must be documented in ADRs (Architecture Decision Records)
- API endpoints must have OpenAPI/Swagger documentation
- Include diagrams for complex systems or workflows
- Maintain a changelog for each release
- Update documentation when code changes
README Files¶
Each module should have a README.md file that includes:
- Purpose of the module
- Installation instructions (if applicable)
- Usage examples
- Configuration options
- Links to relevant documentation
Testing Requirements¶
Unit Tests¶
- Minimum 80% code coverage for all new code
- Use pytest for Python tests
- Test both success and failure paths
- Use mocks and stubs for external dependencies
- Tests should be independent and idempotent
Integration Tests¶
- Test interactions between components
- Include API endpoint tests
- Test database interactions
- Test LLM integration points
Performance Tests¶
- Benchmark critical paths
- Test memory usage for large datasets
- Test concurrent access patterns
Test Naming Convention¶
Example: test_process_memory_item_with_invalid_data_raises_error
Security Guidelines¶
- Follow the OWASP Top 10 security guidelines
- Use parameterized queries for database operations
- Validate and sanitize all user inputs
- Use proper authentication and authorization
- Store secrets securely using environment variables or a secrets manager
- Regularly update dependencies to patch security vulnerabilities
- Implement rate limiting for API endpoints
- Use HTTPS for all communications
- Apply the principle of least privilege
Performance Considerations¶
- Profile code for performance bottlenecks
- Use appropriate data structures for the task
- Consider memory usage, especially for large datasets
- Use caching where appropriate
- Optimize database queries
- Consider asynchronous processing for long-running tasks
- Implement pagination for large result sets
Version Control Practices¶
Branching Strategy¶
- Use a feature branch workflow
- Branch naming convention:
<type>/<issue-number>-<short-description>
- Types: feature, bugfix, hotfix, refactor, docs
- Example:
feature/123-implement-working-memory
- Main branches:
main
: Production-ready codedevelop
: Integration branch for features
Commit Messages¶
Follow the Conventional Commits specification:
Example: feat(memory): implement working memory expiration mechanism
Pull Requests¶
- Link to related issues
- Include a clear description of changes
- Update documentation if necessary
- Ensure all tests pass
- Address code review comments
Code Review Process¶
- All code must be reviewed before merging
- At least one approval is required
- Reviewers should check for:
- Code quality and adherence to standards
- Test coverage
- Documentation
- Security considerations
- Performance implications
- Use constructive feedback
- Authors should respond to all comments
Dependency Management¶
- Use Poetry for Python dependency management
- Pin dependencies to specific versions
- Document the purpose of each dependency
- Regularly audit and update dependencies
- Minimize the number of dependencies
- Consider the license of each dependency
Error Handling¶
- Use specific exception types
- Handle exceptions at the appropriate level
- Log exceptions with context
- Provide helpful error messages
- Don't expose sensitive information in error messages
- Return appropriate HTTP status codes for API errors
try:
result = process_data(input_data)
except ValidationError as e:
logger.warning(f"Validation error: {e}", extra={"input_data_id": input_data.id})
return {"error": "Invalid input data", "details": str(e)}, 400
except DatabaseError as e:
logger.error(f"Database error: {e}", extra={"input_data_id": input_data.id})
return {"error": "Internal server error"}, 500
Logging Standards¶
- Use the standard logging module
- Define appropriate log levels:
- DEBUG: Detailed information for debugging
- INFO: Confirmation that things are working as expected
- WARNING: Something unexpected happened, but the application can continue
- ERROR: A more serious problem that prevented an operation from completing
- CRITICAL: A serious error that might prevent the application from continuing
- Include context in log messages
- Use structured logging
- Don't log sensitive information
logger.info(
"Processing memory item",
extra={
"item_id": item.id,
"memory_tier": item.tier,
"operation": "process",
}
)
Accessibility¶
- Follow WCAG 2.1 AA standards for web interfaces
- Provide alternative text for images
- Ensure keyboard navigation
- Use semantic HTML
- Test with screen readers
- Maintain sufficient color contrast
Internationalization and Localization¶
- Use gettext for internationalization
- Externalize user-facing strings
- Support right-to-left languages
- Format dates, times, and numbers according to locale
- Test with different locales
Continuous Integration and Deployment¶
- Run tests on every pull request
- Enforce code style and linting
- Generate and publish documentation
- Build and test Docker images
- Deploy to staging environment for verification
- Use blue/green deployments for production
Monitoring and Observability¶
- Implement health check endpoints
- Use structured logging
- Collect and analyze metrics
- Set up alerts for critical issues
- Monitor resource usage
- Track error rates and performance
These standards are subject to change as the project evolves. Suggestions for improvements are welcome through the standard pull request process.
Last updated: 2023-11-01