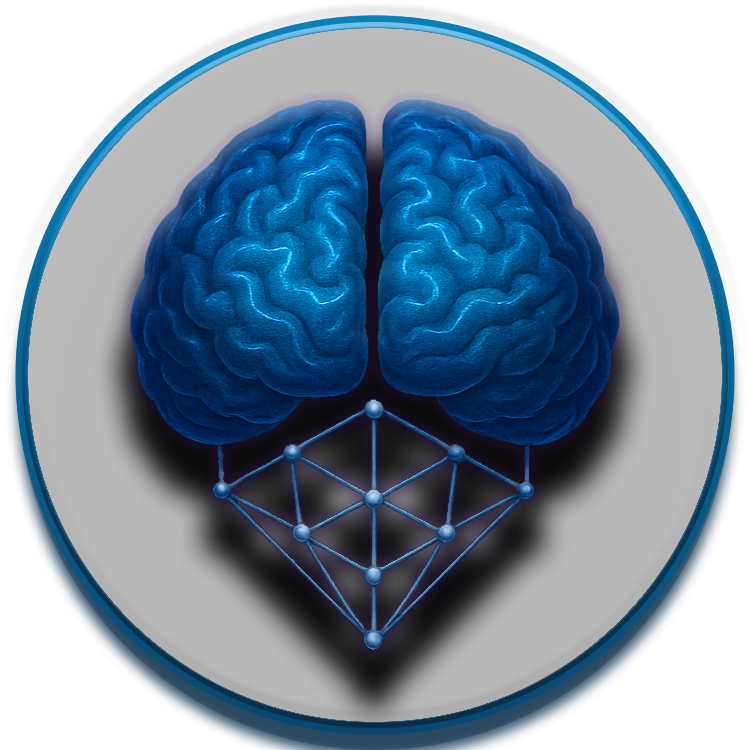
Contributing to NeuroCognitive Architecture (NCA)¶
Thank you for your interest in contributing to the NeuroCognitive Architecture (NCA) project! This document provides guidelines and instructions for contributing to make the process smooth and effective for everyone involved.
Table of Contents¶
- Code of Conduct
- Getting Started
- Project Setup
- Development Environment
- Development Workflow
- Branching Strategy
- Commit Guidelines
- Pull Request Process
- Package Building and Distribution
- Building the Package
- Testing Installation
- Publishing to PyPI
- Coding Standards
- Python Style Guide
- Documentation Guidelines
- Testing Requirements
- Architecture Guidelines
- Review Process
- Issue Reporting
- Feature Requests
- Community Communication
- License
Code of Conduct¶
We are committed to providing a welcoming and inclusive environment for all contributors. Please read and follow our Code of Conduct.
Getting Started¶
Project Setup¶
-
Fork the repository: Start by forking the main repository to your GitHub account.
-
Clone your fork:
-
Add upstream remote:
Development Environment¶
-
Install dependencies: We use Poetry for dependency management:
-
Set up pre-commit hooks:
-
Configure environment variables:
-
Docker environment (optional but recommended):
Development Workflow¶
Branching Strategy¶
We follow a modified GitFlow workflow:
main
: Production-ready codedevelop
: Integration branch for featuresfeature/*
: New features or enhancementsbugfix/*
: Bug fixeshotfix/*
: Urgent fixes for productionrelease/*
: Release preparation
Always create new branches from develop
unless you're working on a hotfix.
Commit Guidelines¶
We follow Conventional Commits for clear and structured commit messages:
Types include: - feat
: New feature - fix
: Bug fix - docs
: Documentation changes - style
: Formatting changes - refactor
: Code refactoring - test
: Adding or modifying tests - chore
: Maintenance tasks
Example:
feat(memory): implement working memory decay function
Add time-based decay to working memory items based on the
forgetting curve algorithm.
Closes #123
Pull Request Process¶
- Create a focused PR: Each PR should address a single concern.
- Update documentation: Include updates to relevant documentation.
- Add tests: Include tests for new functionality or bug fixes.
- Ensure CI passes: All tests, linting, and other checks must pass.
- Request review: Assign reviewers once your PR is ready.
- Address feedback: Respond to all review comments and make necessary changes.
- Squash commits: Before merging, squash commits into logical units.
Package Building and Distribution¶
The NeuroCognitive Architecture (NCA) is distributed as a Python package. This section provides guidelines for building, testing, and publishing the package.
Building the Package¶
We use Poetry for package management and building:
# Ensure your poetry.toml and pyproject.toml are properly configured
# Increment version in pyproject.toml according to semantic versioning
# Build the package (creates both wheel and sdist)
poetry build
Alternatively, you can use the standard Python build tools:
This will create distribution packages in the dist/
directory.
Testing Installation¶
Before publishing, test the installation of your package locally:
# Create a temporary virtual environment
python -m venv .venv-test-pkg
# Activate the environment
# On Windows:
.\.venv-test-pkg\Scripts\activate
# On Unix/MacOS:
source .venv-test-pkg/bin/activate
# Install the package from the local build
pip install dist/*.whl # or dist/*.tar.gz for the source distribution
# Test basic import
python -c "import neuroca; print('Successfully imported neuroca')"
# Deactivate when done
deactivate
Publishing to PyPI¶
Only maintainers with appropriate permissions should publish to PyPI:
# Ensure you have TestPyPI and PyPI credentials configured
# First, test with TestPyPI
poetry config repositories.testpypi https://test.pypi.org/legacy/
poetry publish --repository testpypi
# If everything looks good on TestPyPI, publish to PyPI
poetry publish
# Alternatively, using twine
pip install twine
twine upload dist/*
Remember to create a Git tag for each published version:
Coding Standards¶
Python Style Guide¶
- We follow PEP 8 with some modifications.
- Maximum line length is 100 characters.
- Use type hints for all function parameters and return values.
- Use docstrings for all modules, classes, and functions.
We use the following tools to enforce standards: - Black for code formatting - isort for import sorting - flake8 for linting - mypy for type checking
Documentation Guidelines¶
- Use Google-style docstrings.
- Document all public APIs, classes, and functions.
- Include examples in docstrings where appropriate.
- Keep documentation up-to-date with code changes.
- Add inline comments for complex logic.
Example:
def process_memory_item(item: MemoryItem, decay_factor: float = 0.5) -> MemoryItem:
"""
Process a memory item with decay based on time elapsed.
Args:
item: The memory item to process
decay_factor: Factor controlling decay rate (0.0-1.0)
Returns:
Processed memory item with updated activation
Raises:
ValueError: If decay_factor is outside valid range
Example:
>>> item = MemoryItem(content="test", activation=1.0, timestamp=time.time()-3600)
>>> processed = process_memory_item(item)
>>> processed.activation < 1.0
True
"""
Testing Requirements¶
- All new code should have corresponding tests.
- Aim for at least 80% test coverage for new code.
- Include unit tests, integration tests, and where appropriate, end-to-end tests.
- Test edge cases and error conditions.
- Use pytest for writing and running tests.
Architecture Guidelines¶
- Follow the domain-driven design principles established in the project.
- Maintain separation of concerns between layers.
- Respect the three-tiered memory system architecture.
- Use dependency injection to make components testable.
- Follow the SOLID principles.
- Document architectural decisions that deviate from the established patterns.
Review Process¶
- All code changes require at least one review before merging.
- Reviewers should check for:
- Correctness
- Test coverage
- Code quality and style
- Documentation
- Performance considerations
- Security implications
- Be respectful and constructive in reviews.
- Address all review comments before requesting re-review.
Issue Reporting¶
When reporting issues, please use the issue templates provided and include:
- A clear, descriptive title
- Steps to reproduce the issue
- Expected behavior
- Actual behavior
- Environment details (OS, Python version, etc.)
- Screenshots or logs if applicable
Feature Requests¶
For feature requests, please:
- Check existing issues and discussions to avoid duplicates
- Use the feature request template
- Clearly describe the problem the feature would solve
- Suggest an approach if you have one in mind
- Be open to discussion and refinement
Community Communication¶
- GitHub Discussions: For general questions and discussions
- Issue Tracker: For bugs and feature requests
- Slack/Discord: For real-time communication (links provided in project README)
- Regular Community Calls: Schedule posted in the README
License¶
By contributing to NeuroCognitive Architecture, you agree that your contributions will be licensed under the project's license. See the LICENSE file for details.
Thank you for contributing to NeuroCognitive Architecture! Your efforts help build a more robust and innovative cognitive architecture for LLMs.