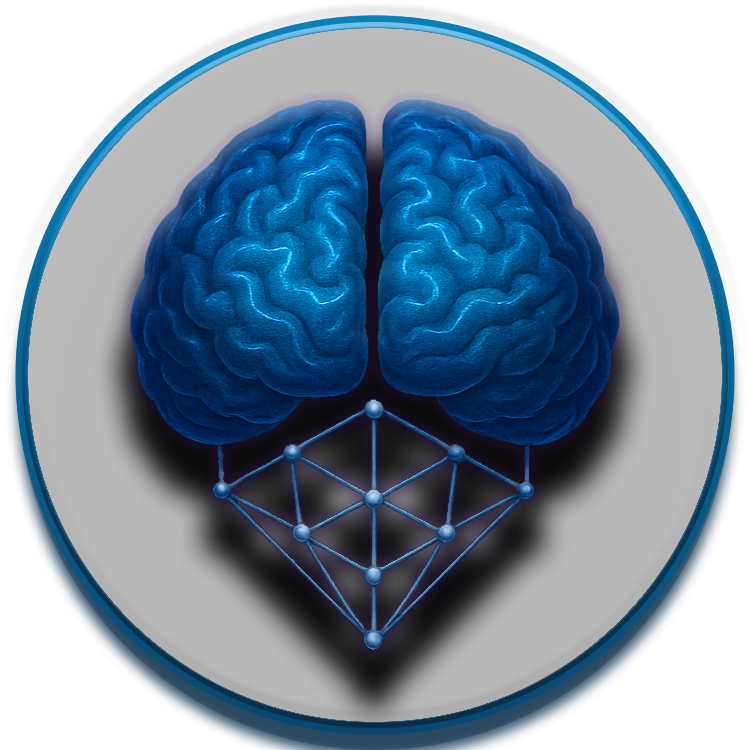
Memory System Architecture Analysis & Refactoring Plan¶
Date: April 14, 2025
Author: Justin Lietz Document Version: 1.0
Executive Summary¶
This document presents a comprehensive analysis of the Neuroca memory system architecture, identifying redundancies, architectural misalignments, and other issues discovered during code refactoring. It outlines a detailed plan for resolving these issues while maintaining system functionality and adhering to AMOS (Apex Modular Organization Standard) guidelines.
The memory system is critical to the Neuroca platform, providing tiered memory storage (STM, MTM, LTM) with human-like forgetting and consolidation mechanisms. However, its evolution has led to multiple implementations of similar functionality, creating maintenance challenges and potential bugs.
We have already taken the first step by refactoring manager.py
into a modular structure. This document outlines the next steps needed to create a cohesive, well-organized memory architecture.
Table of Contents¶
- Current Architecture Analysis
- Redundancy Assessment
- Completed Refactoring
- Recommended Improvements
- Implementation Plan
- Backwards Compatibility
- Risk Assessment
Current Architecture Analysis¶
High-Level Components¶
The current memory system architecture consists of the following main components:
- Tier-Specific Storage Implementations
src/neuroca/memory/stm/storage.py
: Short-Term Memory storagesrc/neuroca/memory/mtm/storage.py
: Medium-Term Memory storage-
src/neuroca/memory/ltm/storage.py
: Long-Term Memory storage -
Memory Type Implementations
src/neuroca/memory/episodic_memory.py
: Episodic memory systemsrc/neuroca/memory/semantic_memory.py
: Semantic memory system-
src/neuroca/memory/working_memory.py
: Working memory system -
Storage Backends
src/neuroca/memory/backends/redis_backend.py
: Redis implementationsrc/neuroca/memory/backends/sql_backend.py
: SQL implementationsrc/neuroca/memory/backends/vector_backend.py
: Vector search implementation-
src/neuroca/memory/backends/factory.py
: Factory for creating backends -
Memory Processes
src/neuroca/memory/memory_consolidation.py
: Memory consolidation functionssrc/neuroca/memory/memory_decay.py
: Memory decay functions-
src/neuroca/memory/memory_retrieval.py
: Memory retrieval functions -
Core Memory System
src/neuroca/core/memory/consolidation.py
: More complex consolidation logicsrc/neuroca/core/memory/episodic_memory.py
: Core episodic memory implementationsrc/neuroca/core/memory/factory.py
: Memory system factorysrc/neuroca/core/memory/health.py
: Memory health monitoringsrc/neuroca/core/memory/interfaces.py
: Memory system interfacessrc/neuroca/core/memory/semantic_memory.py
: Core semantic memory implementation-
src/neuroca/core/memory/working_memory.py
: Core working memory implementation -
Manager (Newly Refactored)
src/neuroca/memory/manager.py
: Original monolithic manager (now a facade)src/neuroca/memory/manager/
directory: Decomposed modules
Component Interactions¶
The interactions between these components reveal several circular dependencies:
- Storage Backend Factory Dependencies:
StorageBackendFactory
inbackends/factory.py
imports from tier-specific storage modules (stm/storage.py
,mtm/storage.py
,ltm/storage.py
)-
These tier-specific modules may also use backends such as Redis, SQL, etc.
-
Memory Consolidation Flow:
-
Multiple implementations of consolidation: simple functions in
memory_consolidation.py
, complex class incore/memory/consolidation.py
, and our new implementation inmanager/consolidation.py
-
Manager Component:
- Previously monolithic implementation now refactored into multiple files
- Uses
StorageBackendFactory
to create storage backends - Implements its own consolidation/decay logic while similar functionality exists elsewhere
Redundancy Assessment¶
Storage Implementation Redundancies¶
Module | Function | Redundant With | Issue |
---|---|---|---|
ltm/storage.py | LTM Storage | sql_backend.py & vector_backend.py | Contains implementations that could be moved to backends |
mtm/storage.py | MTM Storage | redis_backend.py | Contains Redis-like functionality with custom implementation |
stm/storage.py | STM Storage | None (unique) | Generally self-contained but should conform to common interfaces |
Memory Process Redundancies¶
Process | Implementations | Issue |
---|---|---|
Consolidation | • memory_consolidation.py • core/memory/consolidation.py • manager/consolidation.py | Three separate implementations with overlapping functionality |
Decay | • memory_decay.py • manager/decay.py | Two implementations of similar functionality |
Memory Retrieval | • memory_retrieval.py • Retrieval methods in storage classes • Retrieval methods in manager | Multiple implementations of retrieval logic |
Core vs. Regular Memory Implementations¶
The core/memory/
directory contains implementations that appear to duplicate functionality in the regular memory/
directory:
Core Implementation | Regular Implementation | Overlap |
---|---|---|
core/memory/episodic_memory.py | memory/episodic_memory.py | Episodic memory functionality |
core/memory/semantic_memory.py | memory/semantic_memory.py | Semantic memory functionality |
core/memory/working_memory.py | memory/working_memory.py | Working memory functionality |
Code Inspection Findings¶
StorageBackendFactory
Analysis:- Creates backends based on tier (STM, MTM, LTM)
- For each tier, uses a tier-specific storage class (
STMStorage
,MTMStorage
,LTMStorage
) - These tier-specific classes have their own backend implementations
-
Creates circular dependencies and confusion about which implementation to use
-
Consolidation Logic Comparison:
memory_consolidation.py
: Simple functions for adding metadata to memories during consolidationcore/memory/consolidation.py
: ComplexStandardMemoryConsolidator
class with activation thresholds, emotional salience, etc.-
manager/consolidation.py
: New implementation focused on automatic consolidation between tiers -
Decay Logic Comparison:
memory_decay.py
: Simple stub implementations for decay calculationmanager/decay.py
: More elaborate implementation with access count, importance weighting
Completed Refactoring¶
We have already completed the following refactoring:
- Decomposed
src/neuroca/memory/manager.py
(>1000 lines) into separate modules: manager/__init__.py
: Package exportsmanager/models.py
:RankedMemory
data classmanager/utils.py
: Helper functions for formatting, relevance calculationmanager/storage.py
: Storage operations across tiersmanager/consolidation.py
: Memory consolidation between tiersmanager/decay.py
: Memory decay and strengtheningmanager/working_memory.py
: Working memory buffer management-
manager/core.py
: MainMemoryManager
class orchestrating everything -
Created a facade in
manager.py
that re-exports the refactored components for backward compatibility
All files now comply with the AMOS 500-line limit while preserving functionality.
Target Architecture¶
Based on our analysis of the current system, we have identified the clear target architecture we want to achieve. This architecture features clean separation of concerns with proper abstraction layers:
- Storage Backends: Low-level database interfaces (Redis, SQL, Vector)
- Handles direct interaction with specific database technologies
- Provides basic CRUD operations optimized for each database type
-
Completely independent of memory logic
-
Memory Tiers: Logical tier-specific behaviors (STM, MTM, LTM)
- Implements tier-specific behaviors (e.g., TTL for STM, priority for MTM)
- Uses the storage backends for persistence
-
Knows nothing about memory types or the manager
-
Memory Manager: Central orchestration layer
- Coordinates operations across all tiers
- Implements cross-tier functionality (consolidation, decay)
- Provides a clean, unified public API
-
Handles context-driven memory retrieval and working memory
-
Memory Types: (Episodic, Semantic, Working)
- Specialized memory implementations
- Use the Memory Manager as their interface to the system
The goal is to create a cohesive, well-structured system without redundancy or circular dependencies, where each component has a clear responsibility and well-defined interfaces.
Implementation Plan¶
Instead of implementing incremental fixes, we will proceed directly to the target architecture. This ensures we avoid temporary solutions and maintain a clear path to our goal. The plan consists of five clear phases:
Phase 1: Detailed Architecture Design (1 week)¶
- Define Core Interfaces
- Task: Create interface definitions for all core components
- Output:
src/neuroca/memory/interfaces/storage_backend.py
: Abstract interface for storage backendssrc/neuroca/memory/interfaces/memory_tier.py
: Abstract interface for memory tierssrc/neuroca/memory/interfaces/memory_manager.py
: Public API for the memory system
-
Details: Define all methods, parameters, return types, and expected behaviors
-
Design Data Models
- Task: Design standardized data models for memory items
- Output:
src/neuroca/memory/models/
directory with Pydantic models -
Details: Create models for memory items, metadata, search criteria, etc.
-
Map Component Interactions
- Task: Create sequence diagrams for key operations
- Output: Detailed sequence diagrams for operations like add/retrieve/search
-
Details: Document how components interact for each operation
-
Define Directory Structure
- Task: Design the final directory structure
- Output: Directory layout documentation
-
Details: Specify where each component will live in the final architecture
-
Create Comprehensive Test Plan
- Task: Design test cases covering all functionality
- Output: Test specifications for each component
- Details: Include unit, integration, and system tests
Phase 2: Implementation of New Core Components (2 weeks)¶
- Implement Storage Backend Interfaces
- Task: Create backend implementations for Redis, SQL, Vector DB
- Output:
src/neuroca/memory/backends/redis_backend.py
src/neuroca/memory/backends/sql_backend.py
src/neuroca/memory/backends/vector_backend.py
-
Approach: Test-driven development, implement one backend at a time
-
Implement Memory Tier Interfaces
- Task: Create tier implementations for STM, MTM, LTM
- Output:
src/neuroca/memory/tiers/stm.py
src/neuroca/memory/tiers/mtm.py
src/neuroca/memory/tiers/ltm.py
-
Approach: Implement tier-specific logic using the backend interfaces
-
Implement Memory Manager
- Task: Create the new MemoryManager implementation
- Output:
src/neuroca/memory/manager/manager.py
-
Details: Implements memory management operations using the tier interfaces
-
Create Unit Tests
- Task: Write comprehensive tests for all new components
- Output:
tests/unit/memory/
directory with test files - Details: Ensure high code coverage and test all edge cases
Phase 3: Migration of Existing Code (1 week)¶
- Identify All Usage Points
- Task: Find all places in the codebase that use memory systems
- Output: Comprehensive list of files to be updated
-
Details: Include exact file locations and line numbers
-
Create Migration Facade
- Task: Build a facade over the new architecture for backward compatibility
- Output: Updated
src/neuroca/memory/manager.py
-
Details: Ensures old code can use the new implementation seamlessly
-
Update Client Code
- Task: Modify all client code to use the new memory manager
- Schedule: Update code in priority order (core→integration→API→tools)
-
Approach: Systematic update of all identified usage points
-
Integration Testing
- Task: Test the updated code with the new memory system
- Output: Integration test results
- Details: Ensure all functionality works as expected with the new implementation
Phase 4: Cleanup and Removal of Old Code (1 week)¶
- Verify No References to Old Code
- Task: Search for imports of deprecated modules
- Output: Confirmation that no code references the old implementations
-
Details: Use code search to verify complete migration
-
Remove Redundant Implementations
- Task: Delete all redundant code
- Files to Remove:
src/neuroca/memory/memory_consolidation.py
src/neuroca/memory/memory_decay.py
src/neuroca/memory/memory_retrieval.py
src/neuroca/core/memory/*
(if fully superseded)src/neuroca/memory/stm/storage.py
(if fully implemented in new architecture)src/neuroca/memory/mtm/storage.py
(if fully implemented in new architecture)src/neuroca/memory/ltm/storage.py
(if fully implemented in new architecture)
-
Approach: Remove files one by one, running tests after each removal
-
Simplify Factory Implementation
- Task: Update
StorageBackendFactory
to use new architecture - Output: Updated
src/neuroca/memory/backends/factory.py
- Details: Eliminate circular dependencies
Phase 5: Documentation and Final Validation (1 week)¶
- Update Documentation
- Task: Create comprehensive documentation for the new architecture
- Output:
- Updated
src/neuroca/memory/README.md
- Architecture documentation
- API reference
- Updated
-
Details: Include examples, best practices, and migration guides
-
Final System Testing
- Task: Run full test suite and perform manual testing
- Output: Test reports and validation results
-
Details: Ensure all functionality works correctly and there are no regressions
-
Performance Benchmarking
- Task: Compare performance of new vs. old implementations
- Output: Performance metrics
-
Details: Ensure the new implementation meets or exceeds performance requirements
-
Code Quality Review
- Task: Perform final code review
- Output: Code quality metrics
- Details: Ensure the code meets all quality standards and AMOS guidelines
Backwards Compatibility¶
To maintain backward compatibility throughout this refactoring:
- Facade Pattern:
- Keep original entry points (
manager.py
) as facades to new implementations -
Proxy calls to new implementations while maintaining original interfaces
-
Adapter Classes:
- Create adapter classes that implement old interfaces but use new implementations
-
Place these in appropriate locations for easy discovery
-
Deprecation Warnings:
- Use standard Python deprecation warnings to alert developers
- Include specific migration paths in warnings
-
Example:
-
Version Support Policy:
- Define how long deprecated interfaces will be supported
- Communicate this policy clearly in documentation
-
Example policy: "Deprecated interfaces will be supported for two minor versions before removal."
-
Documentation:
- Provide clear migration guides for each deprecated component
- Include code examples showing old vs. new usage
Risk Assessment¶
Risk | Impact | Likelihood | Mitigation |
---|---|---|---|
Breaking existing functionality | High | Medium | Comprehensive test suite, gradual rollout, facade pattern |
Circular dependencies | Medium | High | Careful refactoring with dependency injection or import indirection |
Performance degradation | Medium | Low | Benchmark key operations before and after changes |
Increased complexity during transition | Medium | High | Detailed documentation, clear migration paths |
Missed usage patterns | High | Medium | Thorough code analysis, engagement with all teams |
Key Risk Areas¶
- Integration with Cognitive Control System:
src/neuroca/core/cognitive_control/
may directly use memory systems-
Need to ensure these interactions are preserved or properly migrated
-
API & External Interfaces:
src/neuroca/api/routes/memory.py
exposes memory functionality-
Must maintain compatibility or provide clear migration path
-
Event System Integration:
src/neuroca/core/events/memory.py
suggests event-based interactions-
Ensure event handling is preserved during refactoring
-
Test Coverage Gaps:
- Need to ensure all functionality has adequate test coverage
- Missing tests could allow regressions during refactoring
Mitigation Strategies¶
- Incremental Approach:
- Refactor one component at a time
- Verify functionality after each change
-
Roll back changes if issues are detected
-
Feature Flags:
- Implement feature flags for new implementations
- Allow gradual rollout and easy rollback
-
Example:
-
Monitoring & Logging:
- Add detailed logging during transition
- Monitor for errors or unexpected behavior
-
Set up alerts for potential issues
-
Stakeholder Communication:
- Keep all teams informed of changes
- Provide clear timelines and expectations
- Solicit feedback throughout the process
This document will be updated as refactoring progresses and additional findings are discovered.