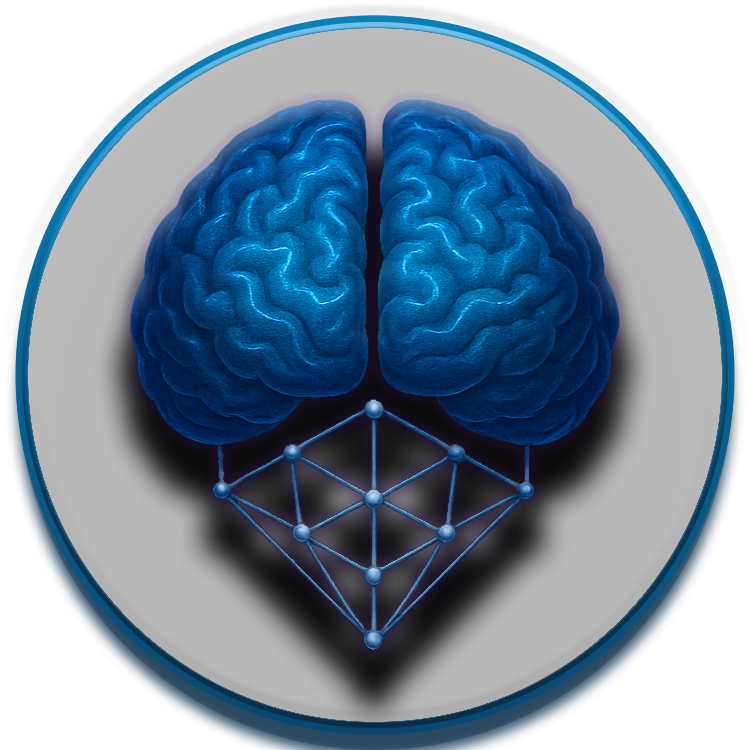
ADR-003: Integration Approach for NeuroCognitive Architecture¶
Status¶
Accepted
Date¶
2023-11-15
Context¶
The NeuroCognitive Architecture (NCA) system requires seamless integration with various Large Language Models (LLMs), external services, and internal components. This integration must be:
- Flexible enough to support multiple LLM providers (OpenAI, Anthropic, open-source models, etc.)
- Resilient to API changes and service disruptions
- Efficient in terms of resource utilization and cost
- Maintainable and extensible as the project evolves
- Secure, with proper handling of credentials and sensitive data
- Compliant with rate limits and usage policies of external services
We need to decide on the architectural patterns, interfaces, and protocols that will govern how NCA components communicate with each other and with external systems.
Decision¶
We will implement a multi-layered integration approach with the following key components:
1. Adapter Pattern for LLM Integration¶
- Create a unified interface (
LLMProvider
) that abstracts away the specifics of each LLM service - Implement concrete adapter classes for each supported LLM provider (e.g.,
OpenAIAdapter
,AnthropicAdapter
,HuggingFaceAdapter
) - Use dependency injection to allow runtime selection of LLM providers
- Include versioning in adapter implementations to handle API evolution
2. Message-Based Communication Architecture¶
- Adopt an asynchronous message-based architecture for internal component communication
- Use a message broker (RabbitMQ) for reliable message delivery between components
- Define standardized message schemas for different types of interactions
- Implement dead-letter queues and retry mechanisms for resilience
3. Circuit Breaker Pattern for External Services¶
- Implement circuit breakers for all external API calls to prevent cascading failures
- Configure appropriate timeouts, retry policies, and fallback mechanisms
- Monitor circuit breaker states as part of system health checks
4. API Gateway for External Access¶
- Create a unified API gateway as the entry point for external systems
- Implement rate limiting, authentication, and request validation at the gateway level
- Version all external APIs to enable non-breaking evolution
5. Event-Driven Architecture for Memory System¶
- Use an event-driven approach for memory tier interactions
- Implement event sourcing for critical memory operations to enable replay and recovery
- Maintain event logs for auditing and debugging purposes
6. Credential and Configuration Management¶
- Store all credentials and configuration in a secure vault (HashiCorp Vault)
- Implement a configuration service that provides runtime configuration to components
- Use environment-specific configuration with appropriate defaults
Consequences¶
Positive¶
- Flexibility: The adapter pattern allows us to easily switch between different LLM providers or use multiple providers simultaneously.
- Resilience: Circuit breakers and message-based architecture improve system stability during partial outages.
- Maintainability: Clear separation of concerns makes the codebase easier to understand and modify.
- Scalability: Asynchronous processing enables better resource utilization and horizontal scaling.
- Future-proofing: The abstraction layers protect against changes in external APIs.
Negative¶
- Complexity: The additional layers and patterns increase the overall system complexity.
- Development overhead: Implementing and maintaining adapters for multiple providers requires ongoing effort.
- Performance considerations: Message-based communication may introduce latency compared to direct calls.
- Testing challenges: The distributed nature of the system makes end-to-end testing more complex.
Neutral¶
- Learning curve: Team members will need to understand the integration patterns and protocols.
- Documentation requirements: Comprehensive documentation of interfaces and message formats will be essential.
Implementation Guidelines¶
- Phased approach:
- Start with a single LLM provider adapter (OpenAI)
- Implement the core message broker infrastructure
- Add circuit breakers to external calls
-
Gradually expand to additional providers and advanced patterns
-
Interface stability:
- Design interfaces with future requirements in mind
- Use semantic versioning for all interfaces
-
Maintain backward compatibility where possible
-
Monitoring and observability:
- Implement detailed logging of all integration points
- Create dashboards for monitoring message flows and circuit breaker states
-
Set up alerts for integration failures and performance degradation
-
Security considerations:
- Encrypt all sensitive data in transit and at rest
- Implement proper authentication for all service-to-service communication
- Regularly audit credential usage and access patterns
Alternatives Considered¶
Direct Integration¶
We considered directly integrating with each LLM provider without abstraction layers. This would be simpler initially but would create tight coupling and make it difficult to switch providers or handle API changes.
Synchronous-Only Communication¶
A fully synchronous architecture would be simpler to implement and debug but would limit scalability and resilience. The chosen message-based approach provides better fault isolation and scaling properties.
Third-Party Integration Platforms¶
We evaluated using third-party integration platforms (e.g., MuleSoft, Zapier) but determined that the custom requirements of our cognitive architecture would be better served by a tailored solution that we control completely.
Related Decisions¶
References¶
- Martin Fowler on the Adapter Pattern
- Circuit Breaker Pattern
- Event-Driven Architecture
- OpenAI API Documentation
- Anthropic API Documentation
Notes¶
This ADR should be reviewed after the initial implementation phase to incorporate lessons learned and adjust the approach as needed. The integration strategy is expected to evolve as the project matures and as the LLM ecosystem continues to develop.