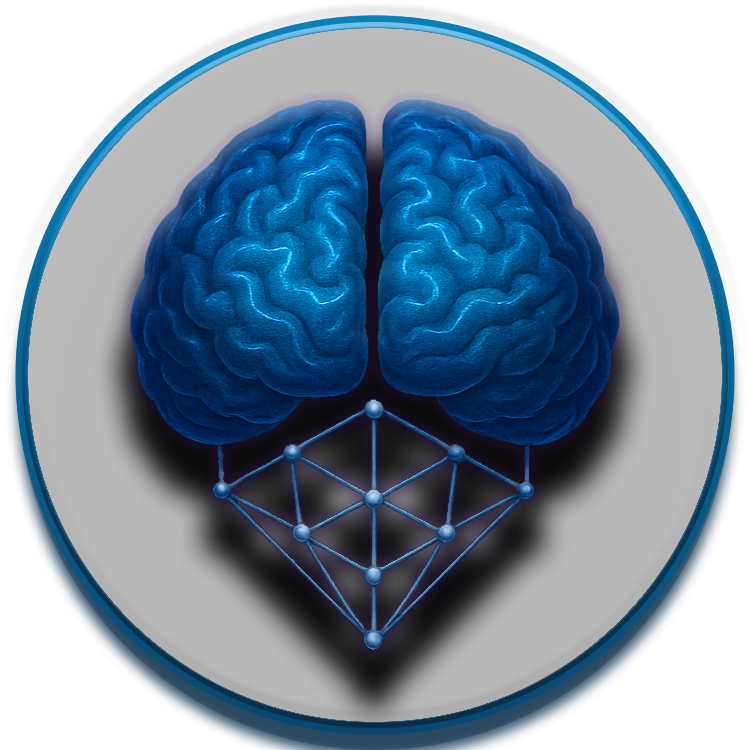
NeuroCognitive Architecture Components¶
Overview¶
This document details the core components of the NeuroCognitive Architecture (NCA) system, their responsibilities, interactions, and implementation details. The NCA is designed as a biologically-inspired cognitive architecture that enhances Large Language Models (LLMs) with multi-tiered memory systems, health dynamics, and cognitive processes that more closely mimic human cognition.
System Components¶
1. Core Cognitive Components¶
1.1 Cognitive Controller¶
The Cognitive Controller serves as the central orchestration mechanism for the NCA system, coordinating interactions between memory systems, health dynamics, and LLM integration.
Key Responsibilities: - Orchestrate information flow between components - Manage cognitive cycles and processing stages - Implement attention mechanisms to prioritize information - Monitor system health and adjust processing accordingly - Coordinate context management across memory tiers
Implementation Details: - Located in core/cognitive_controller.py
- Implements the Observer pattern to monitor component states - Uses a priority queue for attention management - Provides hooks for extensibility and custom cognitive processes
1.2 Perception Module¶
The Perception Module processes incoming information, extracting relevant features and preparing data for cognitive processing.
Key Responsibilities: - Parse and normalize input from various sources - Extract semantic features from text inputs - Identify entities, relationships, and concepts - Prepare information for memory encoding - Apply attention filters based on current cognitive state
Implementation Details: - Located in core/perception/
- Leverages NLP preprocessing techniques - Implements feature extraction pipelines - Uses configurable attention thresholds
2. Memory Systems¶
2.1 Working Memory¶
Working Memory provides temporary storage for information currently being processed, maintaining the active context for cognitive operations.
Key Responsibilities: - Maintain current context and active information - Support manipulation of information for reasoning - Implement capacity constraints and decay mechanisms - Facilitate information transfer between memory tiers - Support parallel processing of multiple information chunks
Implementation Details: - Located in memory/working_memory/
- Implements a graph-based representation for relational information - Uses time-based decay mechanisms - Maintains activation levels for information chunks - Provides interfaces for context manipulation
2.2 Episodic Memory¶
Episodic Memory stores experiences and events with temporal context, enabling recall of specific situations and their details.
Key Responsibilities: - Store experiences with temporal and contextual metadata - Support retrieval based on similarity and relevance - Implement consolidation processes from working memory - Manage memory strength and decay over time - Support narrative construction from episodic sequences
Implementation Details: - Located in memory/episodic_memory/
- Uses vector embeddings for semantic representation - Implements temporal indexing for sequence reconstruction - Provides similarity-based retrieval mechanisms - Supports memory consolidation during idle periods
2.3 Semantic Memory¶
Semantic Memory stores factual knowledge, concepts, and their relationships independent of specific experiences.
Key Responsibilities: - Maintain a knowledge graph of concepts and relationships - Support inference and reasoning over knowledge - Implement abstraction mechanisms from episodic experiences - Provide structured access to factual information - Support knowledge consistency and contradiction resolution
Implementation Details: - Located in memory/semantic_memory/
- Implements a knowledge graph with typed relationships - Uses ontological structures for knowledge organization - Provides reasoning capabilities through graph traversal - Supports incremental knowledge updates
3. Health Dynamics System¶
3.1 Health Monitor¶
The Health Monitor tracks the system's internal state variables and manages the overall health dynamics.
Key Responsibilities: - Track and update health parameters (energy, stress, etc.) - Implement homeostatic mechanisms - Trigger adaptive responses to health state changes - Log health metrics for analysis - Provide interfaces for health state queries
Implementation Details: - Located in core/health/monitor.py
- Implements a publish-subscribe pattern for state changes - Uses configurable thresholds for state transitions - Provides visualization interfaces for health metrics - Supports serialization for persistence
3.2 Adaptation Engine¶
The Adaptation Engine modifies system behavior based on health states and environmental conditions.
Key Responsibilities: - Adjust cognitive parameters based on health states - Implement coping strategies for suboptimal conditions - Manage resource allocation across components - Optimize performance under varying conditions - Learn from past adaptations to improve future responses
Implementation Details: - Located in core/health/adaptation.py
- Uses rule-based and learning-based adaptation strategies - Implements feedback loops for adaptation effectiveness - Provides extensible framework for custom adaptation strategies
4. LLM Integration¶
4.1 LLM Interface¶
The LLM Interface provides standardized communication with underlying language models.
Key Responsibilities: - Abstract LLM-specific implementation details - Manage prompt construction and context windows - Handle token limitations and optimization - Implement caching and batching strategies - Support multiple LLM providers
Implementation Details: - Located in integration/llm_interface/
- Implements adapter patterns for different LLM providers - Uses template-based prompt construction - Provides retry and fallback mechanisms - Implements context window management strategies
4.2 Cognitive Augmentation¶
The Cognitive Augmentation module enhances LLM capabilities with NCA-specific cognitive processes.
Key Responsibilities: - Inject memory contents into LLM context - Implement reasoning frameworks beyond base LLM capabilities - Manage cognitive biases and heuristics - Support metacognitive processes - Enhance output quality through post-processing
Implementation Details: - Located in integration/cognitive_augmentation/
- Implements reasoning frameworks (e.g., chain-of-thought) - Uses memory retrieval augmentation techniques - Provides metacognitive monitoring capabilities - Supports output verification and refinement
5. API and Interface Components¶
5.1 REST API¶
The REST API provides HTTP-based access to NCA capabilities.
Key Responsibilities: - Expose NCA functionality through standardized endpoints - Implement authentication and authorization - Manage rate limiting and resource allocation - Support asynchronous operations for long-running processes - Provide documentation and client libraries
Implementation Details: - Located in api/rest/
- Implements OpenAPI specification - Uses FastAPI for high-performance async operations - Provides comprehensive error handling and validation - Implements security best practices
5.2 WebSocket Interface¶
The WebSocket Interface enables real-time communication with the NCA system.
Key Responsibilities: - Support streaming responses and updates - Maintain persistent connections for interactive sessions - Implement pub/sub mechanisms for event notifications - Manage connection lifecycle and error handling - Support binary and text-based message formats
Implementation Details: - Located in api/websocket/
- Uses standardized WebSocket protocols - Implements heartbeat mechanisms for connection health - Provides authentication and session management - Supports message compression for efficiency
Component Interactions¶
Memory Flow¶
- Perception → Working Memory:
- Incoming information is processed by the Perception Module
- Relevant features are extracted and structured
-
Information is encoded into Working Memory with activation levels
-
Working Memory → Episodic Memory:
- Experiences in Working Memory are consolidated into Episodic Memory
- Temporal context and metadata are attached
-
Consolidation occurs based on importance, novelty, and emotional salience
-
Episodic Memory → Semantic Memory:
- Repeated patterns across episodes are abstracted into semantic knowledge
- Relationships between concepts are extracted and stored
-
Factual information is separated from specific experiences
-
Memory Retrieval Flow:
- Retrieval cues activate relevant information across memory tiers
- Working Memory is populated with retrieved information
- The Cognitive Controller manages retrieval priority and relevance
Health Dynamics Flow¶
- Environment → Health Monitor:
- External conditions affect health parameters
- Internal processes consume resources
-
Health Monitor tracks and updates state variables
-
Health Monitor → Adaptation Engine:
- Health state changes trigger adaptation responses
- Adaptation Engine selects appropriate strategies
-
System parameters are adjusted accordingly
-
Adaptation Engine → Components:
- Memory capacity and decay rates are adjusted
- Attention thresholds are modified
- Resource allocation is optimized
LLM Integration Flow¶
- NCA → LLM Interface:
- Memory contents are selected for context inclusion
- Prompts are constructed with cognitive guidance
-
Requests are optimized for token efficiency
-
LLM Interface → Cognitive Augmentation:
- Raw LLM outputs are processed
- Reasoning is verified and enhanced
- Outputs are integrated with memory systems
Extension Points¶
The NCA architecture provides several extension points for future development:
- Custom Memory Implementations:
- Alternative storage backends
- Specialized memory structures for domain-specific applications
-
Enhanced retrieval mechanisms
-
Additional Health Parameters:
- Domain-specific health variables
- Custom homeostatic mechanisms
-
Specialized adaptation strategies
-
Cognitive Process Extensions:
- Custom reasoning frameworks
- Domain-specific attention mechanisms
-
Specialized learning processes
-
Integration Adapters:
- Support for additional LLM providers
- Integration with external knowledge sources
- Connections to specialized tools and services
Implementation Considerations¶
Performance Optimization¶
- Memory operations use efficient indexing for fast retrieval
- Health dynamics calculations are optimized for minimal overhead
- LLM interactions are batched and cached where appropriate
- Asynchronous processing is used for non-blocking operations
Scalability¶
- Memory systems support sharding for distributed storage
- Processing components can be scaled horizontally
- Resource-intensive operations support parallel execution
- Configuration allows for resource allocation based on deployment environment
Security¶
- All external interfaces implement proper authentication and authorization
- Memory contents are protected with appropriate access controls
- Health state information is secured against unauthorized access
- LLM interactions follow security best practices for prompt injection prevention
Conclusion¶
The component architecture described in this document provides a comprehensive framework for implementing the NeuroCognitive Architecture. By following this design, developers can create a system that enhances LLM capabilities with biologically-inspired cognitive processes, multi-tiered memory, and adaptive health dynamics.
The modular design allows for incremental implementation and testing, supporting the phased development approach outlined in the project roadmap. Each component has clear responsibilities and interfaces, enabling collaborative development while maintaining system coherence.